Programming: ESP8266
Check out my other articles / projects:
- ESP8266 as a Web Server
- ESP8266 Access Point configuration GUI
- ESP8266 / OLED Calendar
- ESP8266 / OLED Date Selector
ESP8266 / OLED Date Selector
This project extends the ESP8266 / OLED Calendar project I developed to simply display a calendar. The date selector, though, adds the ability to select a date by selecting the various components - day, month and year - using a rotary selector. The program is presented as a simple function that allows the calling program to pass a specified date (typically today's date) and returns the selected date. The user has the option to cancel the selection process in which case the original date is returned in the absence of any selection.
![]() |
Visit my GitHub repository to view the project and retrieve the latest code, notify me of errors and so forth. |
Background
The inspiration for this project came from rydepier's Arduino-and-OLED-Calendar project which was targeted at the Arduino and used a different library to drive the OLED. While rewriting it for the ESP8266, I used none of the original code.
This project relies on two separate GitHub projects :
- PaulStoffregen for his Time project and
- Squix78 for his esp8266-oled-ssd1306 library for these little OLED displays.
In Operation
The code snippet below shows just how simple it is to call this helper function. Line 001 sets the current 'system' time to the 2nd September, 2016. Line 002 initialises a time_t variable and invokes the date selector routine using the current date as the starting or default date.
001 002 003 004 005 006 007 008 009 010 |
setTime(12, 0, 0, 2, 9, 2016); time_t t = inputDate(now()); display.clear(); display.setColor(WHITE); display.setTextAlignment(TEXT_ALIGN_LEFT); display.drawString(0, 30, String(year(t)) + "-" + String(month(t)) + "-" + String(day(t)) + " selected."); display.display(); |
The function in action:
Prototype using a KY-040 Rotary Encoder
My prototype uses a KY-040 Rotary Encoder. These use three GPIO pins for input and I have (arbitrarily) used D5, D6 and D7 (GPIO 14, 12 and 13 respectively. These are defined in the constants section of the code and can easily be changed to three unused inputs if they clash with other hardware.
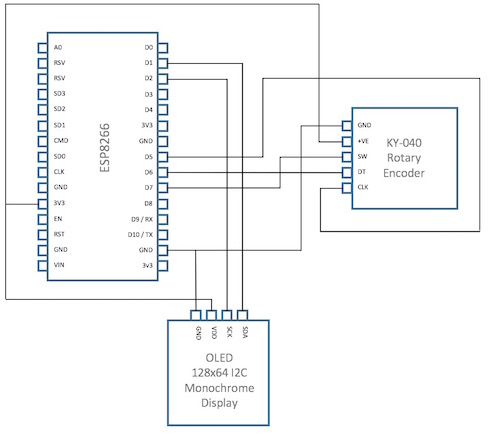

The prototype looks really messy due to it supporting analogue buttons and the KY-040 Rotary Encoder. I have put the encoder on a fly lead so I can use it as a sort of joystick!
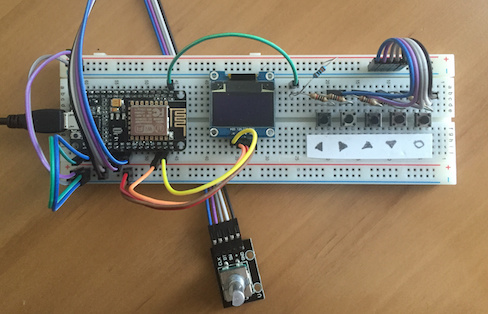
